Starting your coding journey with Python: a helpful guide for beginners
What is Python and how can you start working with it? This article focuses on the basic concepts of the language, such as functions, modules, data structures, libraries, and frameworks, and highlights best practices of writing code.
The author of this article is EPAM senior software engineer Vaibhavi Deshpande.
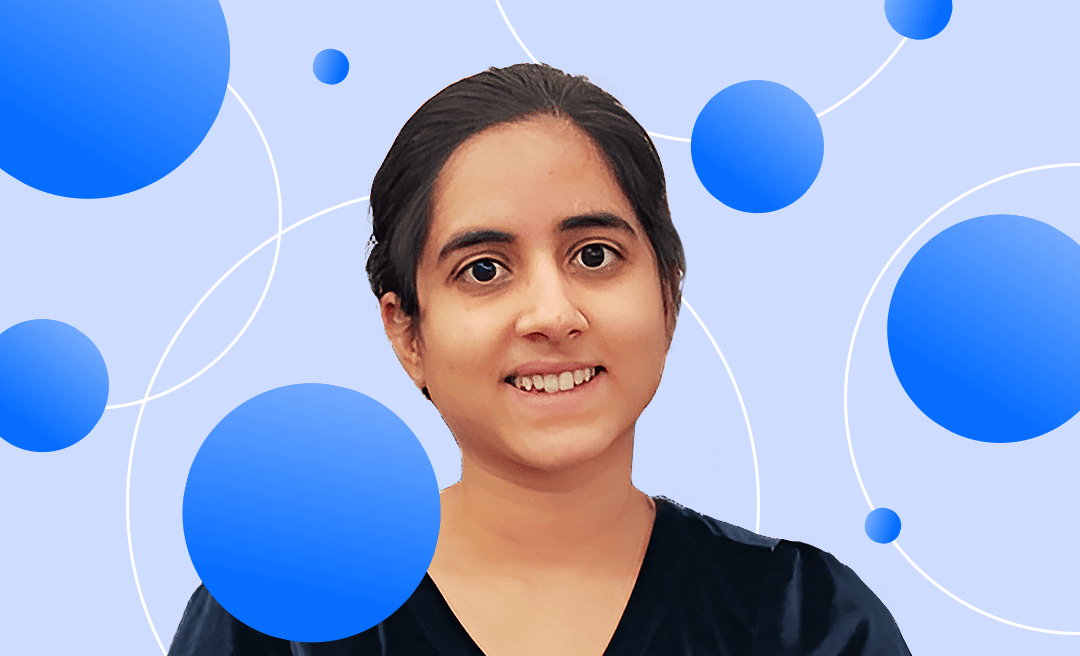
Introduction to Python
Python is a high-level, interpreted programming language that is used for a wide range of applications. It was first released in 1991, and has since become one of the most popular programming languages in the world. Now, let me explain what I meant by “high level” language and “interpreted” language.
What is high-level language and why is Python considered an interpreted language?
- High-level languages are “programming languages that are designed to allow humans to write computer programs and interact with a computer system without having to have specific knowledge of the processor or hardware that the program will run on.“
- Python executes the command line by line and so it is considered as interpreted language.
Python can be used for a variety of applications, including web development, data analysis, scientific computing, machine learning, and more. Many popular web frameworks, such as Django and Flask, are built using Python. It is also used extensively in data science and machine learning, with libraries like NumPy, Pandas, and TensorFlow.
In the image below, you can see how Python has become more popular year over year, and why you must learn it:
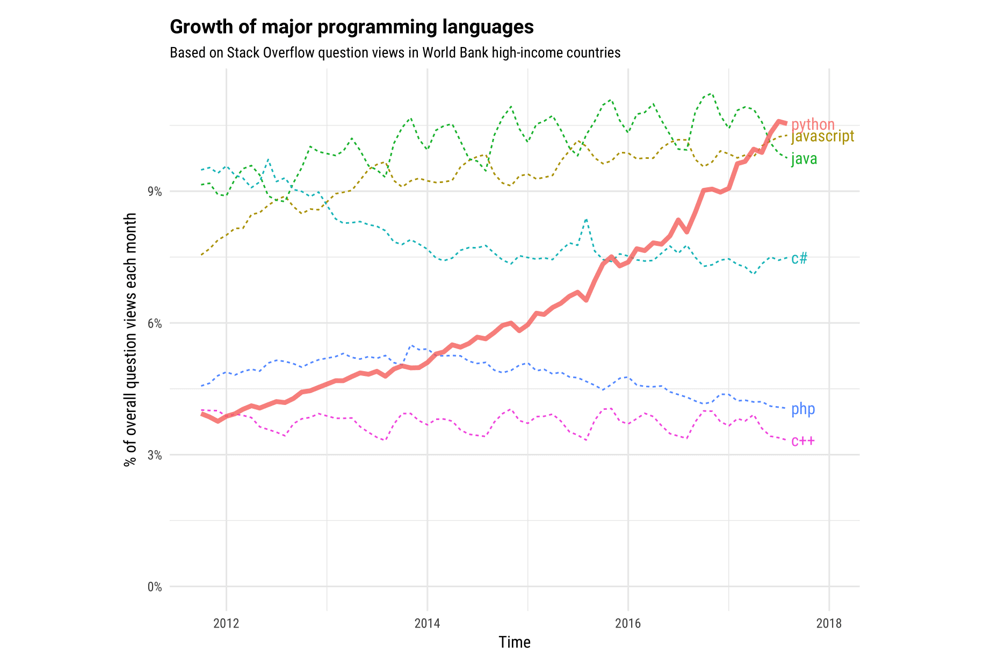
Installation and setup
The first step to get started with Python is to download and install it on your computer. You can download Python from the official website, python.org. Make sure that you download the version of Python that is compatible with your operating system.
In addition to Python, you will also need a text editor or an integrated development environment (IDE) to write your Python code. Popular choices include Visual Studio Code, PyCharm, and Sublime Text.
Here are some links that can help you with the installation and setup of Python:
Python fundamentals
Basic syntax
Python's syntax is straightforward and easy to understand. It uses whitespace indentation instead of curly braces to delimit code blocks, making the code more readable. Here is an example of a simple "Hello, World!" program in Python:
Python supports several data types, including integers, floats, booleans, and strings. It also has built-in control structures like if-else statements, loops, and functions.
Here’s one example of using control structures:
Functions and modules
Functions are an essential part of any programming language, and Python is no exception. You can define your functions in Python using the "def" keyword. Here is an example of a simple function that adds two numbers:
Modules are used to organize your code and reuse code from other sources. Python has a vast library of built-in modules that you can use in your programs. You can also create your modules and import them into your code using the "import" keyword.
Object-oriented programming
Python is an object-oriented programming language, which means that you can create classes and objects to model real-world entities. OOP allows you to build complex applications by organizing your code into logical units. Here is an example of a simple class in Python:
Data structures and algorithms
Python has built-in data structures such as lists, tuples, and dictionaries, which you can use to store and manipulate data. It also has several built-in algorithms, including sorting and searching, that you can use in your programs. Here is an example of how to create a list, tuple, and dictionary in Python:
File handling and input/output
Python supports various file formats and provides built-in functions for file input/output operations. You can open a file in Python using the "open" function and read or write to it using various file methods. Here is an example of how to read a file in Python:
Libraries and frameworks
Python has an extensive library of third-party modules and frameworks that you can use to simplify and speed up your development process. Some popular libraries include NumPy for scientific computing, Pandas for data analysis, and Matplotlib for data visualization. Popular web frameworks built using Python include Django and Flask. Some of the libraries and frameworks, with a brief description of each, are:
- Matplotlib — A popular plotting library in Python that provides a variety of visualization tools to help you better understand your data.
- Tensorflow — A powerful machine learning library that provides a range of tools and techniques for building and training deep neural networks.
- Scikit-learn — A comprehensive library for machine learning that provides tools for data preprocessing, model selection, and evaluation.
- Pygame — A library for game development in Python that provides tools for graphics, sound, and input handling.
- SQLAlchemy — A library for working with databases in Python that provides an Object-Relational Mapping (ORM) tool for managing data.
- Flask-RESTful — A lightweight framework for building RESTful APIs in Python that allows you to quickly create APIs and handle requests and responses.
- Keras — A high-level neural networks API, written in Python and capable of running on top of TensorFlow, that makes it easy to build and experiment with deep learning models.
- Plotly — A visualization library that provides interactive plots and charts for exploring and presenting data.
- Django Rest Framework — A powerful and flexible toolkit for building Web APIs in Django.
- BeautifulSoup — A library for web scraping in Python that allows you to extract data from HTML and XML files.
Each of these libraries and frameworks has its own set of features and capabilities, so it's important to evaluate them based on your specific needs and goals.
Writing code in Python: best practices
Now that we have covered the basics of Python programming, let's talk about some best practices for writing clean, efficient, and maintainable code.
- Use descriptive variable names: Use variable names that clearly describe their purpose. Avoid single-letter variable names or vague names that can make your code difficult to understand.
- Follow the PEP 8 style guide: PEP 8 is a style guide for Python code that promotes consistency and readability. Following this style guide can make your code more readable and maintainable.
- Write modular code: Break your code into smaller, reusable modules. This makes it easier to maintain and test.
- Avoid hardcoding values: Avoid hardcoding values in your code. Instead, use variables or configuration files to store these values. This makes it easier to modify your code without having to search for and change hardcoded values.
- Write unit tests: Write unit tests for your code to ensure that it works as expected. This can catch errors early and save you time in the long run.
- Use version control: Use a version control system like Git to track changes to your code. This makes it easier to collaborate with others and roll back changes if necessary.
- Document your code: Add comments and docstrings to your code to explain what it does and how to use it. This can make it easier for others to understand and use your code.
- Keep your code simple: Write code that is easy to understand and maintain. Avoid unnecessary complexity or clever tricks that can make your code harder to understand.
- Optimize only when necessary: Only optimize your code when necessary. Premature optimization can lead to code that is more complex and harder to maintain.
- Continuously learn and improve: Keep learning and improving your Python skills. Attend conferences, read books and blogs, and work on projects, including Python projects for your resume, to improve your skills and stay up-to-date with the latest developments in the Python community.
Conclusion
Python is a powerful programming language that can be used for a wide range of applications, including web development, data science, machine learning, and more. In this article, we covered the basics of Python programming, including installation and setup, basic syntax, functions and modules, object-oriented programming, data structures and algorithms, file handling and I/O, and libraries and frameworks.
We also discussed some best practices for writing clean, efficient, and maintainable Python code. By following these best practices and continuing to learn and improve your skills, you can become a proficient Python programmer and build great applications.
Resources for further learning and practice:
- Official Python documentation;
- Python.org;
- Codecademy;
- Coursera;
- edX;
- Python for Everybody;
- DataCamp;
- Learn Python the Hard Way;
- Real Python;
- Python Weekly.
I hope these resources help you in your Python learning journey, good luck!