Exploring Java Generic Concepts with Java 8’s Powerful Features
The author of this article is EPAM Lead Software Engineer, Jai Ludhani.
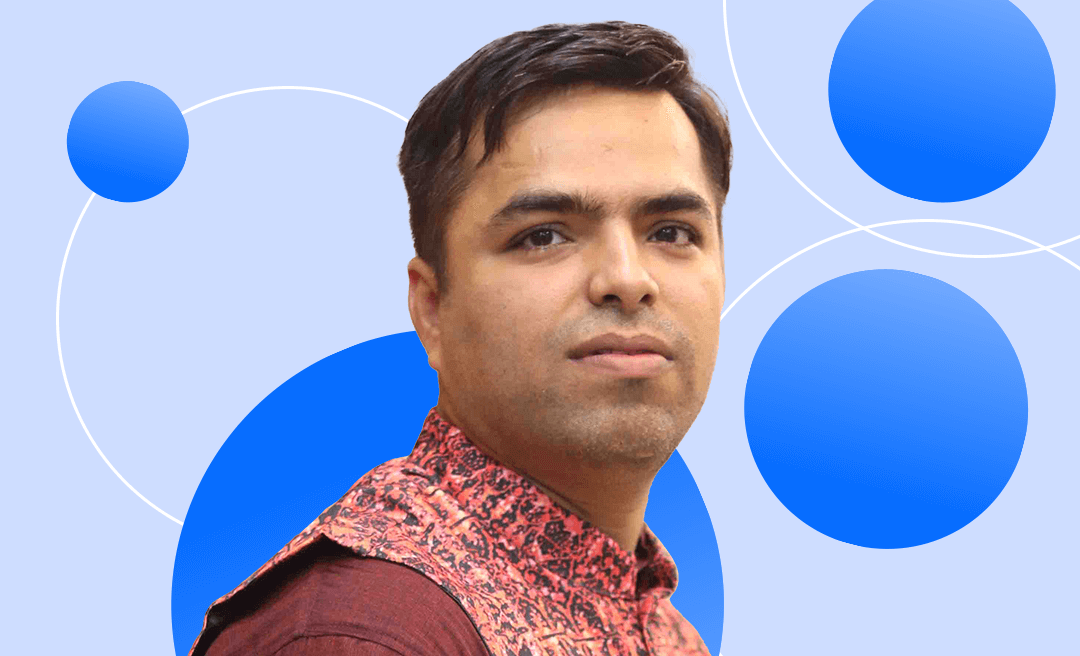
What is Java generics?
Java generics is a powerful feature introduced in Java 5 that allows developers to write code that is more flexible and reusable.
Java generics provides a way to create classes, interfaces, and methods that operate on objects of various types, while maintaining type safety at compile-time. The key benefit is that this enables developers to write generic code that can work with different data types, reducing the need for explicit type casting.
In this article, I explore the main Java generic concepts and demonstrate how Java 8 features can help to make code more concise.
Java generic concepts
1. Generic classes and interfaces
Let's begin with the basics — generic classes and interfaces. They are defined using angle brackets <T>, where 'T' is a placeholder for the actual type.
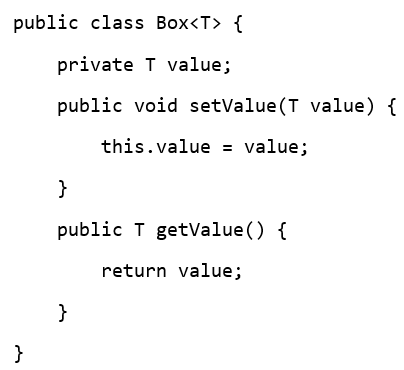
This allows us to create instances of the box class with different types:

2. Generic methods
Similarly, methods can also be generic. This is useful when you want to write a method that operates on different types.
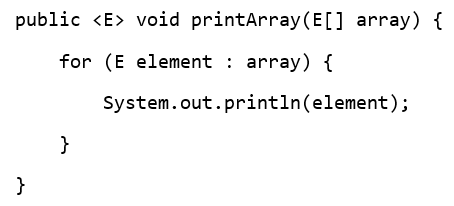
3. Bounded type parameters
Bounded type parameters restrict the types that can be used as arguments in generics. For example, you might want to ensure that a generic type extends to a specific class or implements an interface.
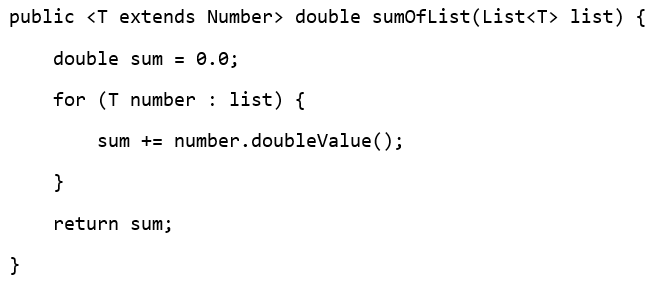
Java 8 features in generics
Java 8 introduced several features that simplify and enhance the use of generics.
1. Lambda expressions
Lambda expressions allow the concise representation of anonymous functions, which is particularly useful in functional interfaces. When working with generics, lambda expressions can be employed to create more expressive and compact code.
2. Stream API
Stream API, introduced in Java 8, allows for the efficient processing of collections. When combined with generics, it enables developers to write expressive and functional code for data manipulation.
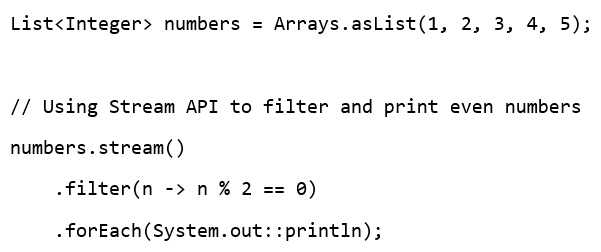
2.1 Generics and the function interface
One of the functional interfaces introduced in Java 8 was the functioninterface, whose abstract method name is apply().
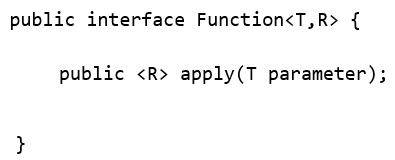
The example below uses the function interface with stream:
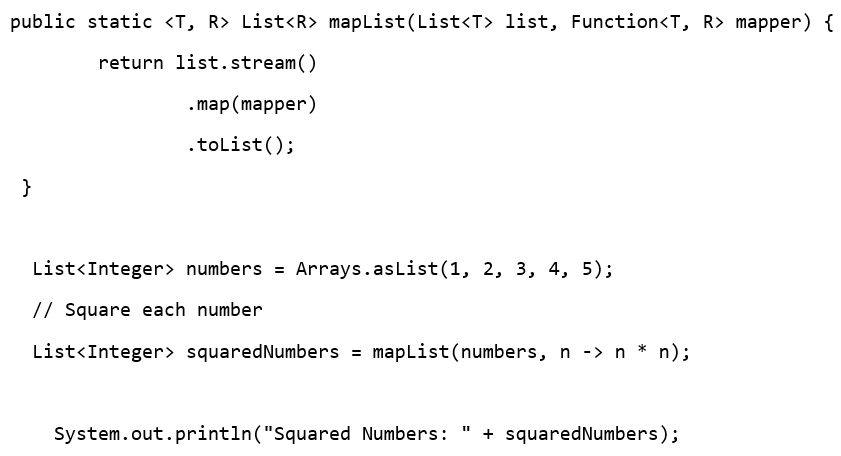
2.2 Generics and the predicate interface
The predicate interface accepts an argument of any type and returns a boolean value. Predicates are often used to filter collections or streams of data.
Below is one example of using the predicate interface:
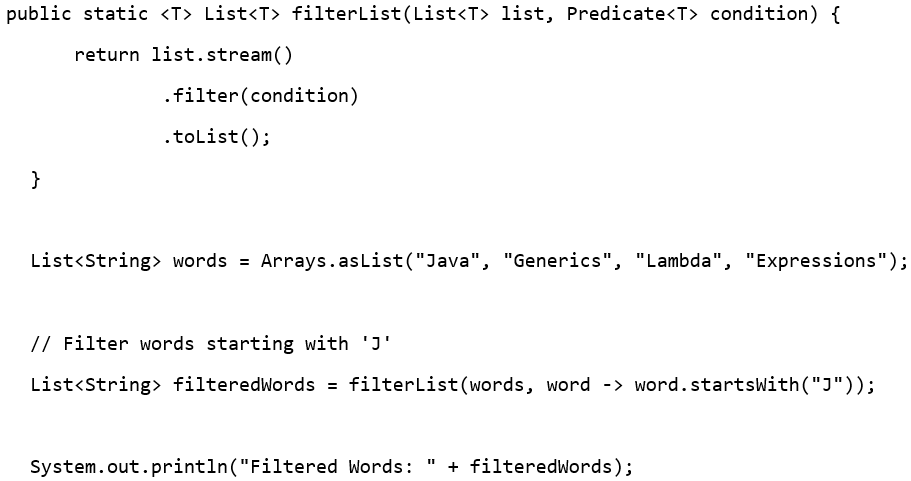
3. Optional
Java 8 also introduced the optional class, which can be used in conjunction with generics to handle the absence of a value more elegantly.
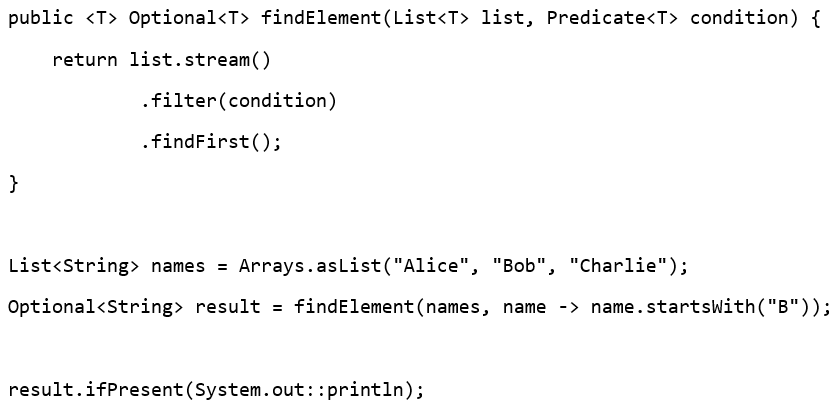
Conclusion
Java generics, coupled with the features introduced in Java 8, offer developers a powerful toolkit for writing flexible, type-safe, and expressive code. By incorporating lambda expressions, Stream API, and optional, developers can create more efficient and concise solutions, making Java a more modern and versatile programming language. Using these features enables developers to write cleaner and more maintainable code, and enhances their overall development experience in Java.