How to Enhance Website Performance with Next.js: 7 Tips and Tricks
The author of this article is EPAM Senior Software Engineer Ravi Kant Singh.
Introduction
A fast and efficient website is crucial for the success of any business. Users expect websites to load quickly and provide a seamless browsing experience.
A powerful tool that can help you meet user expectations is Next.js, a popular framework for building server-side rendered React applications. In this article, I explain how to enhance website performance with Next.js based on my own experience.
1. Server-side rendering (SSR)
One of the key features of Next.js is its ability to perform server-side rendering (SSR). SSR allows the initial page load to be rendered on the server side and sent to the client as HTML, reducing the page loading time. This can significantly improve the performance of your website, especially for content-heavy pages.
By leveraging SSR, Next.js can fetch data from external APIs and databases on the server side and render the page with the data already populated. This eliminates the need for additional API calls on the client side, resulting in faster load times. Additionally, SSR can improve SEO since search engines can easily crawl and index the server-rendered pages.
To implement SSR in Next.js, you can use the getServerSideProps function. This function is used within a page component and allows you to fetch data at the request time before rendering the page.
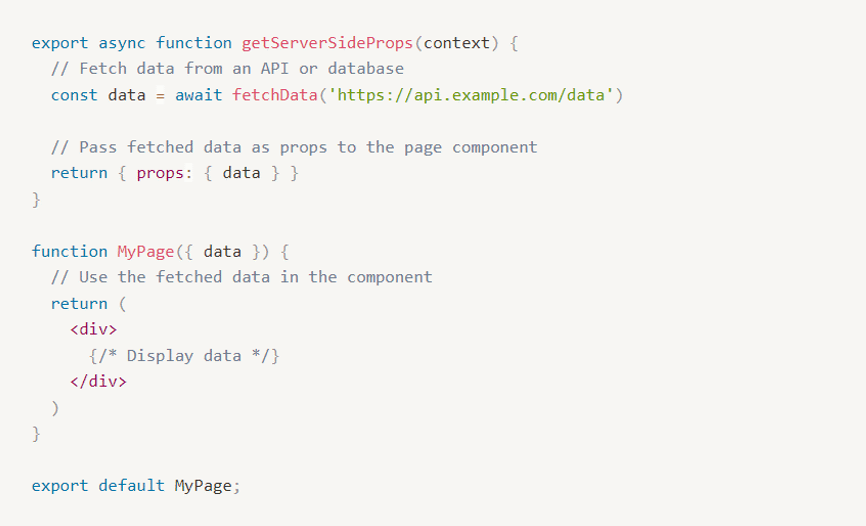
You should use getServerSideProps only if you need to render a page for which data must be fetched at the request time.
2. Static site generation (SSG)
Static site generation in Next.js pre-builds HTML pages at build time. That means that the pages are generated during the build process and serve as static files when requested. This approach results in faster page loads since the content is already prepared and doesn't require server-side processing for each request.
To use static site generation in Next.js, you can use the getStaticProps function. This function allows you to fetch data at build time. You should also use getStaticProps if the data required to render the page is available at build time ahead of a user’s request.
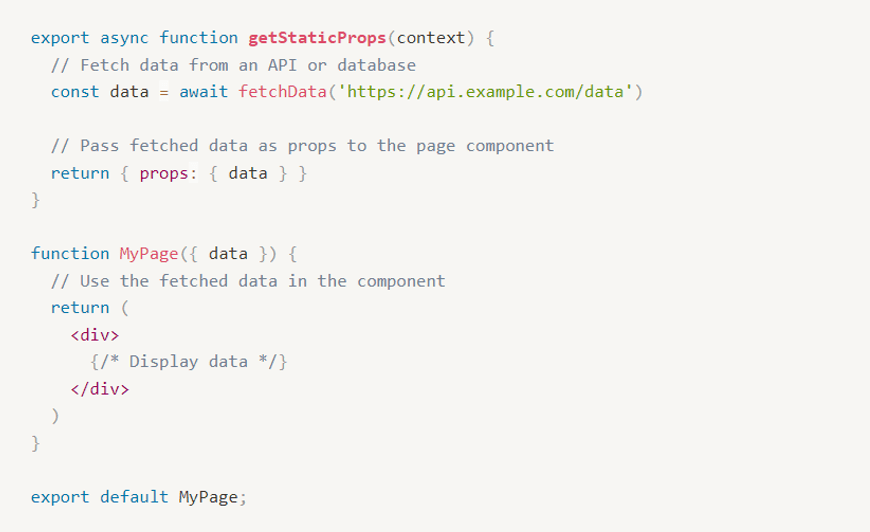
3. Code splitting and lazy loading
Two other techniques to enhance website performance with Next.js are code splitting and lazy loading. Code splitting allows you to divide your JavaScript code into smaller chunks that can be loaded on demand as a user navigates your website. This prevents the user from downloading unnecessary code upfront, resulting in faster initial page loads.
Next.js provides built-in support for code splitting, making it easy to implement. Using dynamic imports and the import() function, you can dynamically load components or modules only when they are needed. This reduces the initial bundle size and improves the overall performance of your website.
Lazy loading is used to defer the loading of non-essential resources (such as images, components, or data) until they are needed. This helps reduce the initial page load time by loading the essential content first and then fetching additional resources as the user interacts with the page.
In the context of React and Next.js, lazy loading is often used to load components asynchronously only when they are required, not at the initial rendering phase.
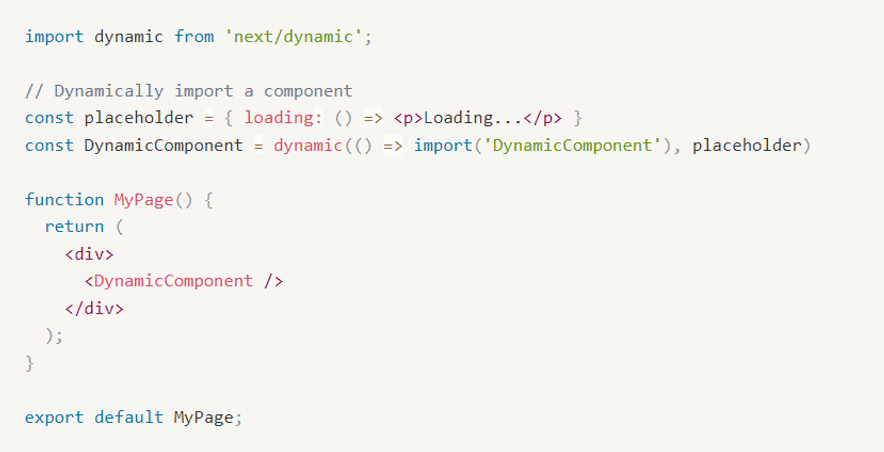
In the example above, the DynamicComponent will be loaded lazily when it's needed. The loading prop in the dynamic function allows you to specify a placeholder component to display while the actual component is being loaded.
By combining code splitting and lazy loading in Next.js, you can optimize the performance of your application by loading the required code and resources only when they are needed, improving the user experience and reducing initial load times.
4. Caching and optimizing assets
Caching and optimizing assets is another great strategy for enhancing website performance. Caching static assets like CSS and JavaScript files enables you to reduce the number of requests made to the server and improve the overall load time of your website.
Next.js provides built-in support for asset optimization and caching. It automatically generates optimized versions of your CSS and JavaScript files, appending a unique hash to the file name. This allows the browser to cache the assets and only fetch them again if there are changes, resulting in faster subsequent page loads.
The benefits of this approach are:
- Minification: Next.js automatically minifies JavaScript and CSS files during the production build process. This reduces file sizes by removing unnecessary characters, such as white spaces and comments, without altering functionality.
- Image optimization: Next.js provides an Image component that automatically optimizes images. You can use this component to serve images in modern formats like WebP and automatically generate multiple image sizes for responsive images.
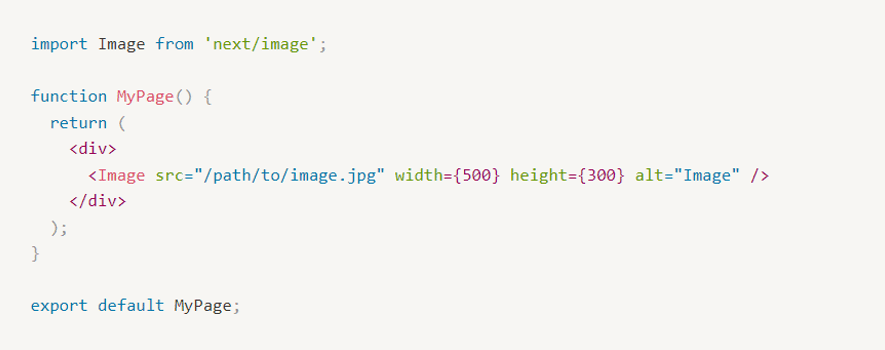
- Bundle size analysis: Analyze and optimize your application's bundle size to identify large dependencies or code chunks that can be split or optimized further. Tools like webpack-bundle-analyzer can help visualize the size of your bundles.
- Service workers: Implementing service workers enables more advanced caching strategies such as the use of the Cache API to control cache storage and retrieval, allowing assets to be served even when offline. Next.js supports service workers, which can be configured in your application.
- HTTP caching headers: Next.js leverages HTTP caching headers to control how browsers and CDNs cache assets. You can set caching headers using the next.config.js file to specify cache-control settings for different types of assets:
In the example above:
- The first entry sets the caching headers for all pages (source: '/(.*)') to cache assets for 1 year
- The second entry targets a specific directory for images (source: '/static/images/(.*)') and sets the cache duration to 30 days.
You can customize these headers based on your specific caching requirements for different types of assets or routes in your Next.js application.
5. Performance monitoring and optimization
In addition to the above-mentioned techniques, it is important to continuously monitor and optimize the performance of your Next.js website. This involves using performance monitoring tools to identify any bottlenecks or areas for improvement.
Tools such as Lighthouse, WebPageTest, and Google Analytics can provide valuable insights into the performance of your website. They can help you identify areas where you can further optimize your code, reduce file sizes, or improve server response times. Regularly monitoring your website performance and making necessary optimizations can lead to significant improvements in load times and overall user experience.
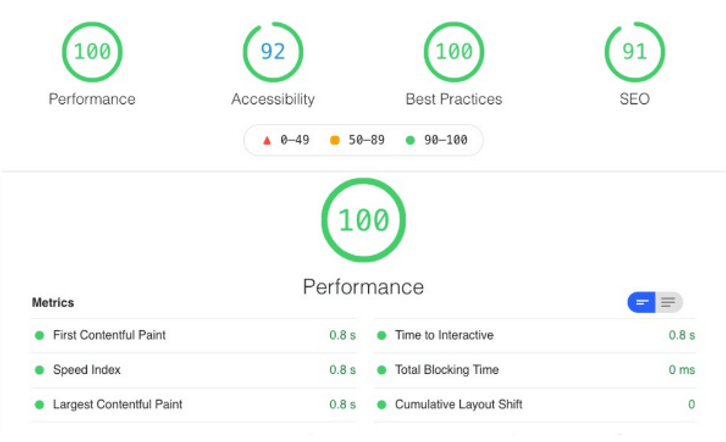
6. Mobile optimization
More and more people use mobile devices for browsing the web, so it is essential to optimize your Next.js website for mobile performance. Mobile optimization involves designing your website to be responsive and load quickly on mobile devices. It helps ensure that your website delivers a fast and seamless browsing experience to users on smartphones and tablets.
Next.js provides built-in support for responsive design, allowing you to create mobile-friendly layouts that adapt to different screen sizes. Additionally, you can optimize your website performance on mobile devices by minimizing the use of large images or videos, reducing the number of HTTP requests, and using browser caching.
7. Continuous performance testing and optimization
Optimizing website performance is not a one-time task, but an ongoing process. It is important to continuously test and optimize performance as new features are added, or changes are made.
Implementing continuous integration and continuous deployment (CI/CD) practices can help automate the testing and optimization process. By incorporating performance testing into your CI/CD pipeline, you can catch any performance issues early and ensure that your website maintains optimal performance throughout its lifecycle.
Conclusion
Next.js offers a range of powerful features and techniques for enhancing website performance. By leveraging server-side rendering, code splitting, lazy loading, asset optimization, and continuous performance testing, you can create a fast and efficient website.
Remember to monitor your website performance regularly, optimize it for mobile devices, and implement CI/CD practices to ensure ongoing improvements. Following these tips will enable you to level up your Next.js website performance and deliver a top-notch user experience.